Client-side data fetching
When there is a web page that shows user-specific data that does not need any optimization for SEO client-side data fetching is the way to go. In Next.js, just as in React.js, you can use the useEffect
hook with async functions to fetch data and the useState hook to display them in the browser. You can also use libraries such as Axios to fetch API data. However, Next.js recommends using SWR( stale-while-revalidate ), a React hooks library for client-side data fetching. The useSWR
is the main hook in this library.
You can do client-side data fetching in components or in pages. But, there is a difference in data-fetching. At the page level, it happens at run-time and updates page content with new data, whereas at the component level, it is done at the time when the component is mounted and the content of the component is updated.
useSWR for the data-fetching
SWR stands for stale-while-revalidate. It consists of React hook
SWR is a scheme that improves asynchronous data fetching. So, does it so that? it will execute as follows
- First, check if there is any data available in the cache ( stale data ), if there is it will return this data
- Then, it will revalidate the data.
- Finally, it sends the updated data
so, how does it do this? with the use of a hook called useSWR
.
How to use useSWR hook
we are going to create a fake dashboard.
First, you need to start and set up a fake REST API in your development environment. I have explained how to do this under the heading “Let’s build an application …” in my post: Ultimate guide to incremental static regeneration(ISR ) in next-js. Please follow the steps from 1 to 6.
After you have set up the JSON server with db.json, add the following JSON object
{
"dashboard":{ "username": "John", "email":"[email protected]" }
}
- after that, create a folder and name it “dashboard”.
- now, create an index.js file inside the “dashboard” folder.
- add the following code to the index.js.
import useSWR from 'swr'
import Link from 'next/link';
const fetcher = (...args) => fetch(...args).then((res) => res.json())
export default function Profile() {
const { data, error } = useSWR('http://localhost:4000/dashboard', fetcher );
if (error) return <div>Failed to load</div>
if (!data) return <div>Loading, please wait!</div>
return (
<div>
<div>Welcome { data.username }</div>
<p>Email: { data.email }</p>
</div>
)
}
4. you can run the app in the development mode with npm run dev
. Note that at this time the JSON server should be running in the background. If you have not done it, you can do so with npm run server
.
5. one you start the application, you can open the browser and access the URL http://localhost:3000/dashboard. you will see that before the browser displays the data, it will display “Loading, please wait!”.
6. Now, you can change data in db.json and see how the data in the browser changes. you do not need to refresh the browser. This is one of the many advantages of using SWR. This particular feature is automatic revalidation. It happens when your current focus is on the browser. If you use the useState
and the useEffect
to implement this application, you have to refresh the browser to see the changes in data.
Note: You can disable automatic revalidation by adding { revalidateOnFocus: false } to useSWR
.
useSWR('http://localhost:4000/dashboard', fetcher, { revalidateOnFocus: false } );
7. Another important aspect to note is that when you build this application ( with npm run build
), only the static part of the index.js is pre-rendered. Therefore, based on the initial state (of not having any data ), NEXT.js will pre-render “Loading, please wait!” as an HTML document. It will not render any file based on data coming from the API. This is, of course, the default behavior of NEXT.js( This has nothing to do with using SWR ).
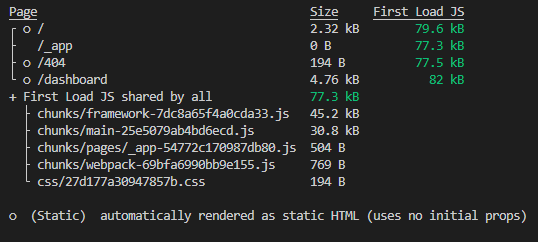
8. You can open the network tab and see the pre-rendered HTML document. ( Make sure to refresh the browser) . If you refresh the browser, you will also see the JSON file. In contrast to SSG with getStatcProps, there is no pre-rendered HTML document with data, which is fine as client-side rendering is for fetching user-specific data with no significance in SEO.
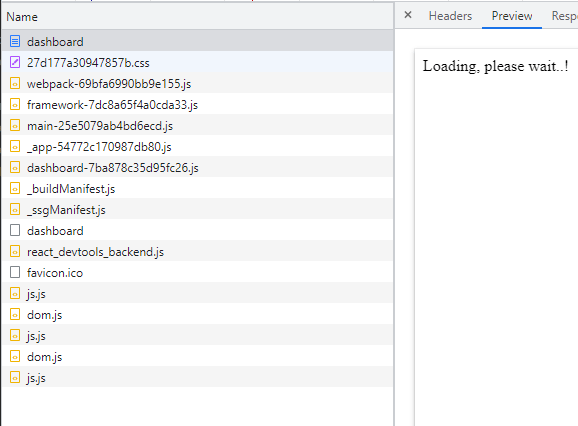
Summary
Client-side data fetching is suitable when you fetch user-specific data such as displaying the user’s dashboard. You can use useState and useEffect to implement in such cases. However, the NEXT.js team recommends the SWR, the React hooks library. The useSWR
is one of the most critical hooks for data fetching with added functionalities such as automatic revalidation.