Table of Contents
What is filtering in JavaScript?
Filtering in JavaScript refers to the process of extracting a subset of elements from a collection based on a specific condition or criteria. It allows you to create a new array or collection that includes only those elements that meet the specified conditions.
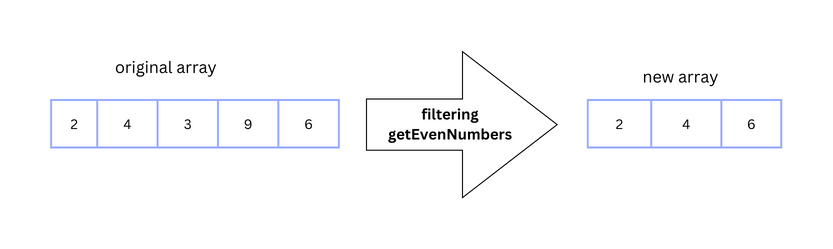
Filtering in Javascript can be achieved by using methods such as find(), map(), flatMap(), and reduce(). However, the most common method used by developers for filtering is the Javascript array filter() method.
What is the JavaScript filter method?
The JavaScript filter()
method is an array method used to create a new array containing elements from the original array that meet a specified condition. It takes a callback function as an argument, which is executed for each element in the array. The callback function should return true
or false
based on whether the element should be included in the resulting array.
syntax: array.filter(callback(element, index, arr ) , thisArg );
array
: The original array on which the filter() method is called.callback
: A function that is executed on each element of the array.element
: The current element being processed in the array.index (optional)
: The index of the current element being processed.arr (optional)
: The original array on which the filter() method is called.thisArg (optional)
: Default undefined. A value passed to the callback function asthis
value
If you remove all these optional parameters, the simplest syntax to use the filter method is
array.filter( callback( element ));
Ex: 1
//original array
const numbers = [1, 2, 3, 4, 5, 6];
//callback function
function getEvenNumbers(num){
return num % 2 === 0
}
//evenNumbers is the new array
const evenNumbers = numbers.filter(getEvenNumbers);
console.log(evenNumbers); // Output: [2, 4, 6]
You can refactor the above code as follows:
const numbers = [1, 2, 3, 4, 5, 6];
//callback function is in the form of an arrow function
const evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4, 6]
Ex 2:
const books = [
{ title: 'The Great Gatsby', author: 'F. Scott Fitzgerald', genre: 'Fiction' },
{ title: 'To Kill a Mockingbird', author: 'Harper Lee', genre: 'Fiction' },
{ title: 'The Catcher in the Rye', author: 'J.D. Salinger', genre: 'Fiction' },
{ title: '1984', author: 'George Orwell', genre: 'Science Fiction' },
{ title: 'Pride and Prejudice', author: 'Jane Austen', genre: 'Fiction' },
{ title: 'The Hobbit', author: 'J.R.R. Tolkien', genre: 'Fantasy' }
];
// Filter books by genre
const fictionBooks = books.filter(book => book.genre === 'Fiction');
console.log(fictionBooks);
/* output
[
{ title: 'The Great Gatsby', author: 'F. Scott Fitzgerald', genre: 'Fiction' },
{ title: 'To Kill a Mockingbird', author: 'Harper Lee', genre: 'Fiction' },
{ title: 'The Catcher in the Rye', author: 'J.D. Salinger', genre: 'Fiction' },
{ title: 'Pride and Prejudice', author: 'Jane Austen', genre: 'Fiction' }
]
*/
How to use the “thisArg” parameter of the filter method
You may have noticed that the filter method takes another optional parameter. What is the use of it? You can use this parameter to explicitly specify the value to be used as this
within the callback function. It sets the context or the value of this
when executing the callback function.
You may use this parameter in 3 circumstances.
Working with object methods
If you have a callback function that references object methods (e.g., this.method()
), setting thisArg to the object ensures that the methods are correctly invoked within the callback function.
Ex:
const obj = {
data: [1, 2, 3, 4, 5],
threshold: 3,
isAboveThreshold(element) {
return element > this.threshold;
}
};
const filteredData = obj.data.filter( obj.isAboveThreshold, obj );
console.log(filteredData); // Output: [4, 5]
In the above example, we have an object obj with a data array and a threshold property. We define a method isAboveThreshold()
that checks if an element is greater than the threshold value.
By passing obj as the thisArg parameter in the filter()
method, we ensure that the this
value within the isAboveThreshold()
method refers to the obj object. This allows us to access the threshold property correctly and filter the data accordingly.
Preserving lexical scope
If you have a callback function that relies on lexical scope (e.g., using variables defined outside the function), setting thisArg allows you to maintain the desired scope within the callback.
function checkLength(element) {
return element.length > this.minLength;
}
const words = ['apple', 'mango', 'cherry', 'durian'];
const minLengthFilter = words.filter(checkLength, { minLength: 5 });
console.log(minLengthFilter ); // Output: ["cherry", "durian"]
In this example, the function checkLength()
checks if the length of an element is greater than the minimum length. By setting { minLength: 5 }
as the thisArg
parameter, we maintain the desired lexical scope within the callback function. The resulting minLengthFilter
array will contain only the words with a length greater than 5.
Utilizing custom context
You can set thisArg
to a specific object or value to use as the context within the callback function, allowing you to access properties or methods specific to that object or value.
function filterEven(element) {
return element % 2 === 0;
}
const numbers = [1, 2, 3, 4, 5, 6];
const customContext = { message: 'Even numbers:' };
const evenNumbers = numbers.filter(filterEven, customContext);
// Output: Even numbers: 2,4,6
console.log(`${customContext.message} ${evenNumbers}`);
In this example, the function filterEven()
checks if an element is even. By setting customContext
as the thisArg
parameter, we utilize a custom context within the callback function. The custom context object contains a message property that we can reference within the callback or outside of it. In this case, we log a message along with the evenNumbers
array to indicate that it contains even numbers.
Wrapping up
Filtering provides a powerful method for selectively processing and extracting data from arrays according to specific criteria. This process is commonly utilized to locate items that match particular conditions, eliminate undesired elements, or construct data subsets that fulfill defined prerequisites. In the realm of JavaScript, the Array.prototype.filter() method emerges as a cornerstone for effective data filtering.
The Array.prototype.filter() function enables the creation of a new array by systematically assessing each element within the original array. It does so through the application of a custom callback function, which receives the element itself, its index, and the array under consideration as parameters. The callback’s true or false return value determines whether an element is included or excluded in the new array. It’s crucial to note that the filter() method maintains the integrity of the original array, generating a fresh array solely comprising elements that adhere to the specified condition. This makes the filter() method a preferred solution when the objective is to extract or filter particular elements from an array while keeping the initial array intact and unaltered.